Note
Click here to download the full example code
Simulate Supernovae¶
Simulating a multiply-imaged supernova.
Create a simulated multiply-imaged supernova that we can then fit,
with no microlensing included in the simulation. Note that your final
printed information will be different, as this is a randomly generated
supernova. The function being used in these examples is
createMultiplyImagedSN()
.
Run this notebook with Google Colab.¶
No Microlensing
import sntd
import numpy as np
myMISN = sntd.createMultiplyImagedSN(sourcename='salt2-extended', snType='Ia', redshift=1.4,z_lens=.53, bands=['F110W','F160W'],
zp=[26.8,26.2], cadence=5., epochs=35.,time_delays=[20., 70.], magnifications=[10,5],
objectName='My Type Ia SN',telescopename='HST',av_host=False)
print(myMISN)
myMISN.plot_object()
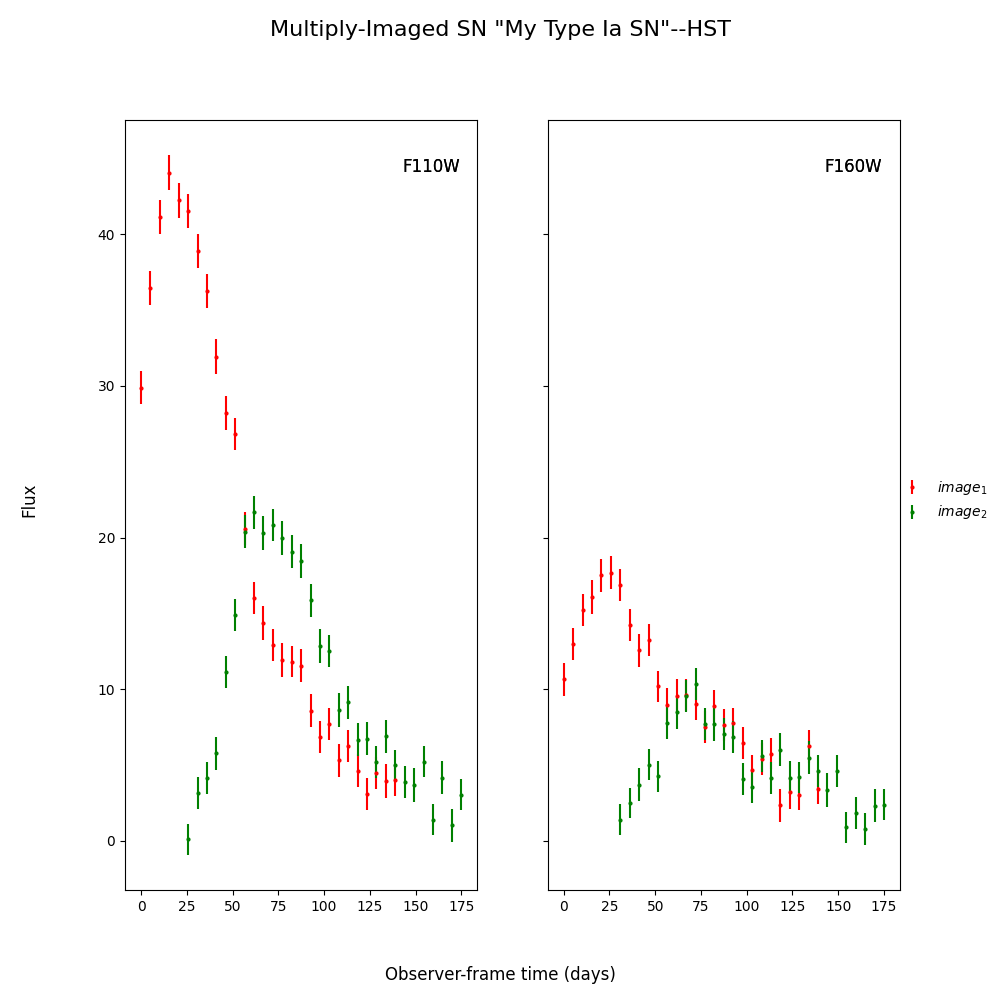
Out:
Telescope: HST
Object: My Type Ia SN
Number of bands: 2
------------------
Image: image_1:
Bands: ['F110W', 'F160W']
Date Range: 0.00000->138.97059
Number of points: 56
Metadata:
z:1.4
t0:20.0
x0:5.9554678275562e-06
x1:1.5139356714340149
c:-0.17709989559397843
sourcez:1.4
hostebv:0
lensebv:0
lensz:0.53
mu:10
td:20.0
------------------
Image: image_2:
Bands: ['F110W', 'F160W']
Date Range: 25.73529->175.00000
Number of points: 59
Metadata:
z:1.4
t0:70.0
x0:2.9777339137781e-06
x1:1.5139356714340149
c:-0.17709989559397843
sourcez:1.4
hostebv:0
lensebv:0
lensz:0.53
mu:5
td:70.0
------------------
<Figure size 1000x1000 with 2 Axes>
Specify the distributions you want to use for any model parameter by providing a function that returns the parameter in any way you want.
def x1_func():
return(np.random.normal(1,.5))
def c_func():
return(np.random.normal(-.05,.02))
param_funcs={'x1':x1_func,'c':c_func}
myMISN2 = sntd.createMultiplyImagedSN(sourcename='salt2-extended', snType='Ia', redshift=1.33,z_lens=.53, bands=['F110W','F125W'],
zp=[26.8,26.2], cadence=5., epochs=35.,time_delays=[10., 70.], magnifications=[7,3.5],
objectName='My Type Ia SN',telescopename='HST',sn_params=param_funcs)
print(myMISN2)
myMISN2.plot_object()
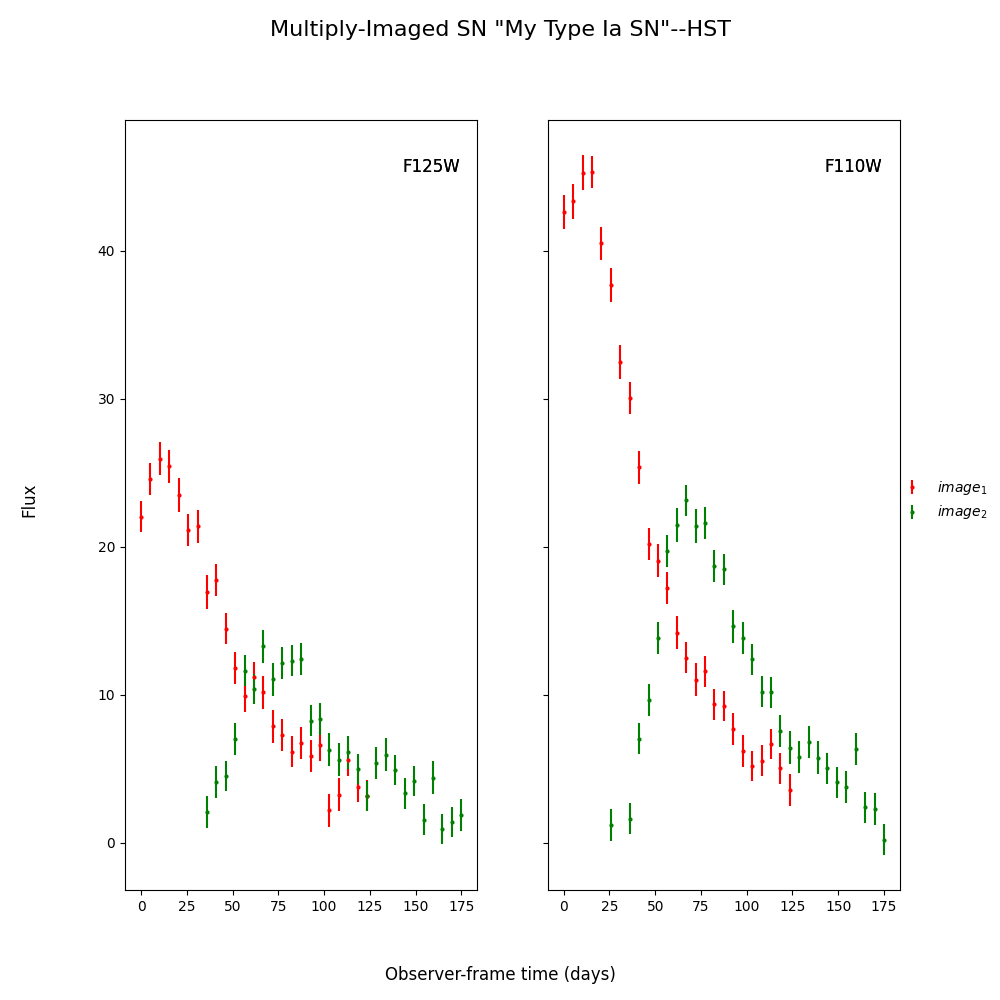
Out:
Telescope: HST
Object: My Type Ia SN
Number of bands: 2
------------------
Image: image_1:
Bands: ['F125W', 'F110W']
Date Range: 0.00000->123.52941
Number of points: 50
Metadata:
z:1.33
t0:10.0
x0:8.380581686942374e-06
x1:1.1026083422743245
c:-0.03916533959272924
sourcez:1.33
hostebv:0.0967741935483871
lensebv:0
lensz:0.53
mu:7
td:10.0
------------------
Image: image_2:
Bands: ['F125W', 'F110W']
Date Range: 25.73529->175.00000
Number of points: 57
Metadata:
z:1.33
t0:70.0
x0:4.190290843471187e-06
x1:1.1026083422743245
c:-0.03916533959272924
sourcez:1.33
hostebv:0.0967741935483871
lensebv:0
lensz:0.53
mu:3.5
td:70.0
------------------
<Figure size 1000x1000 with 2 Axes>
Specify the distributions you want to use for dust parameters by providing a function that returns the parameter in any way you want.
def hostav_func():
return(np.random.normal(.5,.1))
def lensav_func():
return(np.random.normal(.7,.2))
param_funcs={'host':hostav_func,'lens':lensav_func}
myMISN3 = sntd.createMultiplyImagedSN(sourcename='salt2-extended', snType='Ia', redshift=1.33,z_lens=.53, bands=['F110W','F125W'],
zp=[26.8,26.2], cadence=5., epochs=35.,time_delays=[10., 70.], magnifications=[7,3.5],
objectName='My Type Ia SN',telescopename='HST',av_dists=param_funcs)
print(myMISN3)
myMISN3.plot_object()
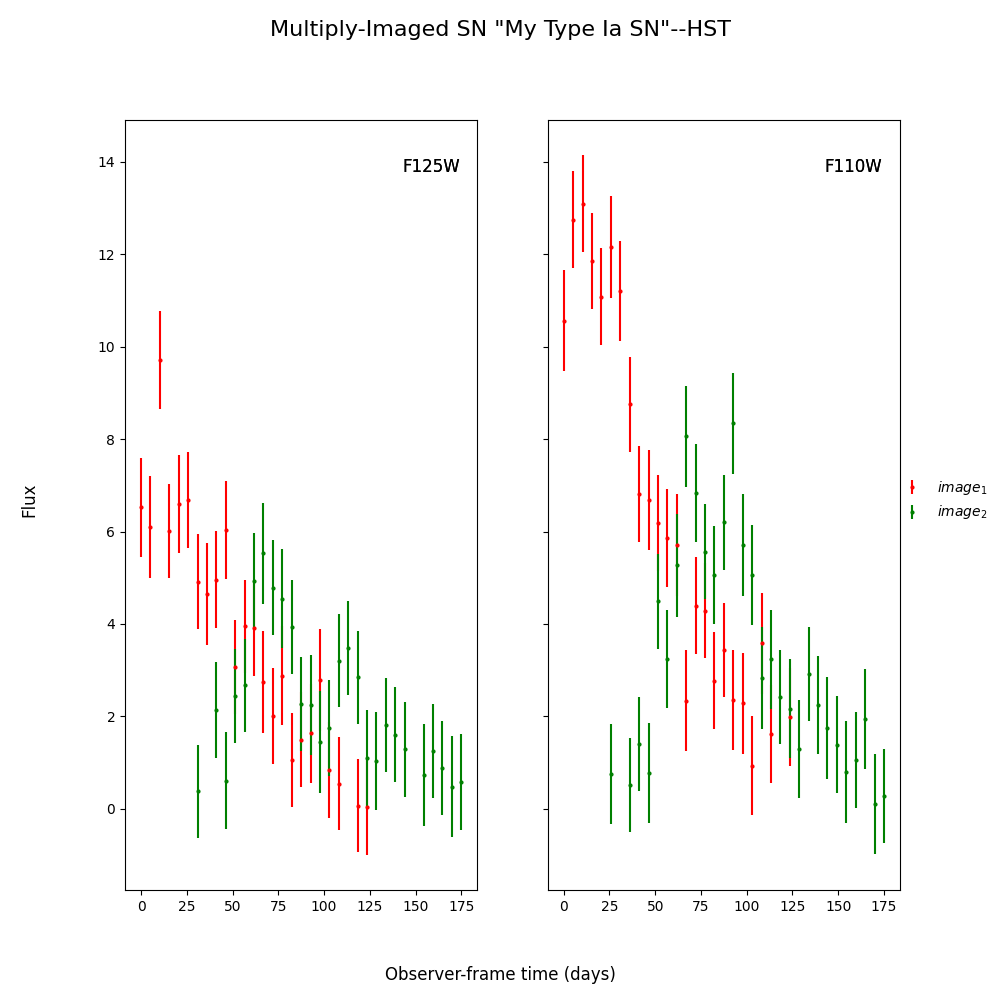
Out:
Telescope: HST
Object: My Type Ia SN
Number of bands: 2
------------------
Image: image_1:
Bands: ['F125W', 'F110W']
Date Range: 0.00000->123.52941
Number of points: 48
Metadata:
z:1.33
t0:10.0
x0:4.354187651106564e-06
x1:1.6358939552410352
c:-0.08458570217749427
sourcez:1.33
hostebv:0.15535413969553127
lensebv:0.18363144965770012
lensz:0.53
mu:7
td:10.0
------------------
Image: image_2:
Bands: ['F125W', 'F110W']
Date Range: 25.73529->175.00000
Number of points: 56
Metadata:
z:1.33
t0:70.0
x0:2.177093825553282e-06
x1:1.6358939552410352
c:-0.08458570217749427
sourcez:1.33
hostebv:0.15535413969553127
lensebv:0.18363144965770012
lensz:0.53
mu:3.5
td:70.0
------------------
<Figure size 1000x1000 with 2 Axes>
Total running time of the script: ( 0 minutes 0.569 seconds)